Image Service
Resizing images for your site on the fly can be a little challenging to do. Storyblok's image service provides you with high-quality images in different formats and sizes for all devices and platforms! This guide will show you how to configure your images, including the format, layout, type, and image processing options.
Contents
- Basic Usage
- Automatic WebP Support Detection
- Optimizing Images
- Resizing
- Fit-in
- Changing Image Format
- Image Quality Optimizations
- Facial Detection and Smart Cropping
- Custom Focal Point
- Grayscale
- Blur
- Rotation
- Flip
- Brightness
- Rounded Corners
- Combining Filters
- Manual Cropping
- Caching in Image Service
- Technical Limits
- Migrating from the Previous Version of Image Service
Basic Usage
You can use Storyblok image service to optimize, resize and crop images for different platforms and devices. To do this, add a /m/
at the end of your asset URL, followed by additional filters and parameters we will learn in this guide.
The image service will transform every image on the Storyblok servers for you and then send it through our CDN to it as fast as possible.
SVG (Scalable Vector Graphics) can't be cropped, resized, or optimized since they can be scaled automatically.
Here's an example image below:
)
Automatic WebP Support Detection
WebP is an image format that is well known for providing better compression compared to other formats. Since smaller images will make your pages load faster, our image service will help you increase your project's speed.
Append /m/
to the end of the image URL, and they will be automatically served in WebP if your browser supports it. You can force a specific format by using the format filter.
Optimize
You can make your images lighter by simply appending /m/
at the end of the URLs. This will enable server-side WebP support detection without performing any other changes to the image.
https://a.storyblok.com/f/39898/3310x2192/e4ec08624e/demo-image.jpeg/m/
https://a-us.storyblok.com/f/1020842/3310x2192/d3ff0ecb29/demo-image.jpg/m/
https://a-ca.storyblok.com/f/2000245/3310x2192/3561e44912/demo-image.jpg/m/
https://a-ap.storyblok.com/f/3000466/3310x2192/49656c0365/demo-image.jpg/m/
The Storyblok Image Service has region-specific base URLs. Select one of the tabs above to check each region-specific URL. We use the European Union endpoint as the example below. You can replace the region-specific base URLs for your use cases.
Resizing
The Storyblok Image Service's resizing feature supports a maximum resolution of 4,000 x 4,000 pixels. For example, if /m/200x5000
is requested, the service will generate an image sized 200 x 4,000 pixels.
Type | Path | Param |
---|---|---|
Original | ||
Resized - Static | /m | /500x500 |
Proportional to Width | /m | /200x0 |
Proportional to Height | /m | /0x200 |
Resizing: Static
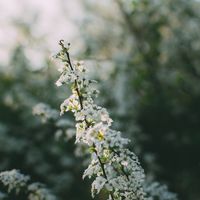

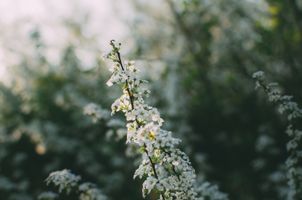
Fit-In
The fit-in argument specifies that the image should not be auto-cropped but auto-resized (shrunk) to fit inside the imaginary width and height box instead.
To fill out the box with a color, use the fill filter filters:fill(CCCCCC)
with a hexadecimal RGB expression (without the #
character). If you want to fill your image with transparent pixels use filter:fill(transparent)
combined with :format(png)
. This might add transparent pixels and make the asset a png image even with .jpeg
as the file extension of your original image.
Type | Path | Param |
---|---|---|
Original | ||
Fit-In with CCCCCC background | /m | /fit-in/200x200/filters:fill(CCCCCC) |
Fit-In with transparent background | /m | /fit-in/200x200/filters:fill(transparent):format(png) |
Fit-In with CCCCCC background
)
))
Changing the Format
Storyblok Image Service supports AVIF in all regions except China.
To change the image format, use the format filter. Supported formats are "webp", "jpeg" "png", and "avif".
Example:
https://a.storyblok.com/f/39898/3310x2192/e4ec08624e/demo-image.jpeg/m/200x0/filters:format(png)
)
Quality Optimization
You can change the compression rate of your JPEG images using the quality
filter. The value can be 0-100
.
When using high values for the quality filter, like quality(100)
the file size increases more than the original file size.
Type | Path | Param |
---|---|---|
Original | ||
10% Quality | /m | /filters:quality(10) |
Resized and 10% Quality | /m | /200x0/filters:quality(10) |
By default, the compression rate (quality
filter) is set to 80.
Resized and 10% Quality
)
Facial Detection and Smart Cropping
When cropping an image, define a smart filter that crops around the focal point. To use, insert the keyword ‘smart’ after the size definition. In our example, a different demo image since flowers don't have a face.
Type | Path | Param |
---|---|---|
Original | ||
Resized without Smart Crop | /m | /600x130 |
Resized with Smart Crop | /m | /600x130/smart |
Resized without Smart Crop


Custom Focal Point
Use the focal point method to define the center of an image, Do this by adding the filter to the URL of the image as shown below:
filters:focal(<left>x<top>:<right>x<bottom>)
Examples:
To focus on the bottom of the image:
https://a.storyblok.com/f/39898/1000x600/d962430746/demo-image-human.jpeg/m/600x130/filters:focal(450x500:550x600)
)
Focus on the top of the image:
https://a.storyblok.com/f/39898/1000x600/d962430746/demo-image-human.jpeg/m/600x130/filters:focal(450x0:550x100)
)
Manual Cropping
You can manually crop specific areas of an image by defining a rectangular cropping area. To do this, declare coordinates for the two points.
The two points of the rectangle are the top-left (first-point) and the bottom-right corners of the rectangle. You have to define the points as pairs of coordinates starting from the top-left corner of the image.
/<left>x<top>:<left>x<top>/
You can also combine cropping with the resizing functionality.
Examples:
To crop a square of 100 pixels starting from the coordinate 300 (x) and 200 (y):
https://a.storyblok.com/f/39898/1000x600/d962430746/demo-image-human.jpeg/m/300x200:400x300/
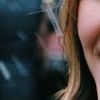
Cropping Around the Focal Point
If you want to crop an area around a focal point in your image. Do this by calculating the coordinates of the top-left and bottom-right.
Given your focal point with coordinates X and Y and the size of the area around your point L, calculate the coordinates of the top left corner and bottom right corner of your cropping area with this method:
- top-left x = X - L/2
- top-left y = Y - L/2
- bottom-right x = X + L/2
- bottom-right y = Y + L/2
Examples:
Your focal point has coordinates 400:300 and you want to crop a square of 100 pixels around it.
- top-left x: 400 - 100/2 = 350
- top-left y: 300 - 100/2 = 250
- bottom-right x: 400 + 100/2 = 450
- bottom-right y: 300 + 100/2 = 350
https://a.storyblok.com/f/39898/1000x600/d962430746/demo-image-human.jpeg/m/350x250:450x350
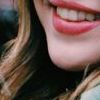
)
Blur
You can blur images with the blur(radius, [sigma])
filter. This is particularly useful for placeholders for lazy-loaded images.
The parameters are:
radius
: An int value between 0 and 150. the higher the number, the more blurred the image will be;sigma
: Optional. Defaults to the same value as the radius. Sigma is used in the gaussian function for blurring.
Examples:
https://a.storyblok.com/f/39898/1000x600/d962430746/demo-image-human.jpeg/m/600x230/filters:blur(10)
)
)
Flip
Flip your images with the resizing parameter. Adding a -
in front of one of the sizes will make the image flip on the same axis. If you don't need to resize your image, use 0
as a value for that axis.
Examples:
Horizontally flipped image without resizing
https://a.storyblok.com/f/39898/1000x600/d962430746/demo-image-human.jpeg/m/-0x0
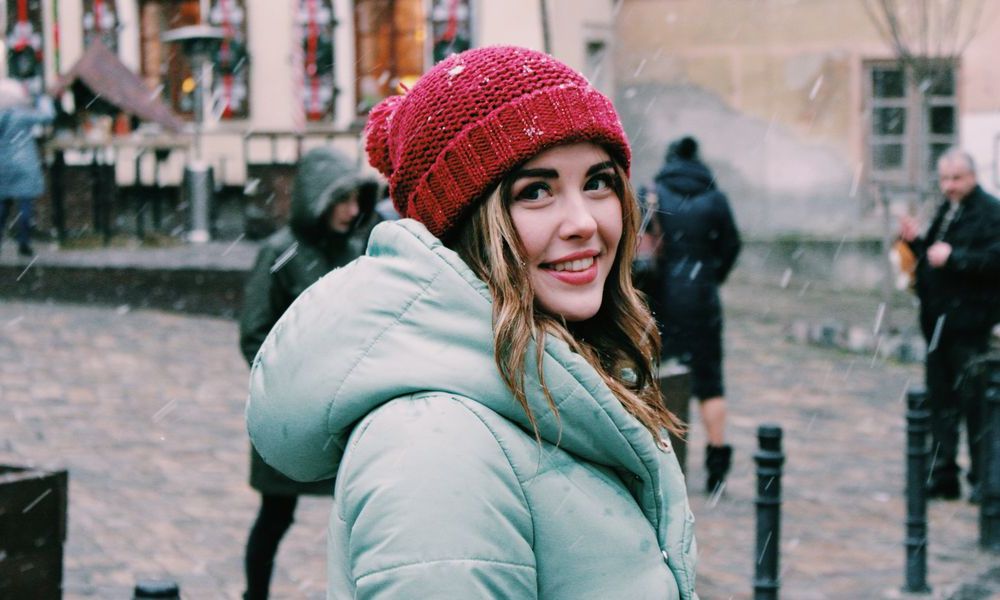
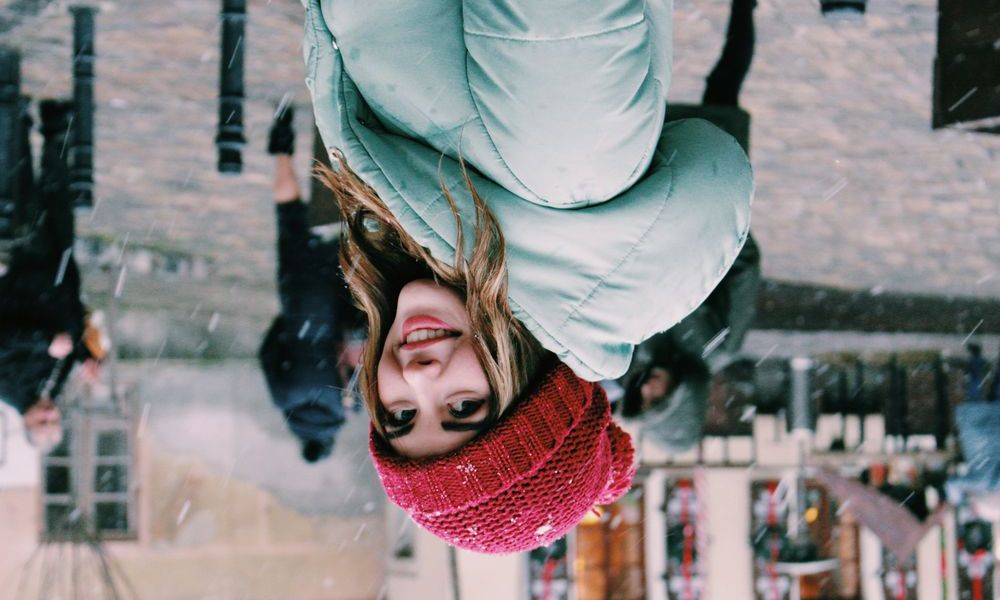

)
Rounded corners
Add rounded corners to your image and define a different radius for each of them with the round_corner(a|b,r,g,b,transparent)
filter.
The parameters are:
- a is an integer representing the radius size;
- b is optional, and it's another parameter for the ellipsis of the rounded corners;
- r, g, b are the background color outside the rounded corners;
- transparent is an optional value from 0 to 1 to set the opacity of the background color.
filters:round_corner(<top-left>,<top-right>,<bottom-right>,<bottom-left>)
Examples:
https://a.storyblok.com/f/39898/1000x600/d962430746/demo-image-human.jpeg/m/600x230/filters:round_corner(30,255,255,255)
)
Caching
With Image service v1, all assets were heavily cached, but with Image service v2, WebP formats are cached automatically.
If you use a special URL to autodetect image WebP format, Storyblok does not cache the asset
By default, assets are not cached if you decide to use the automatic WebP detection. To cache, you need to add a URL path + "Accept" in your image header. If the Accept: WebP header exists in the request, the image service will convert to WebP if it does not remain in the original format (The browser is responsible for adding this header)
Once a cache is created using the accept header, we can have several versions of the cache by different browser versions. But after a request, the cache will already be created. The server will check what image has to be served and if it exists, and then it'll deliver it if it's already there or generate it and deliver it. You can also use a specific file type and cache your asset as Storyblok is always caching, although we do not generate the images every time, even with WebP autodetection.
Technical Limits
Using Storyblok's Image Service is subject to technical limits. A complete overview is provided in the developer guide on Technical Limits.
Migrating from the previous version of the service
If you are still using the previous version of the image service and are happy with that, keep using it. Anyway, we strongly advise you to migrate to the new version because you can benefit from the automatic WebP support detection, which will let you deliver lighter images to the users of your platform.
The new version of our Image Service supports avif convention along with WebP.
Migrating to the new version is very simple because you just need to:
- change back the domain
img2.storyblok.com
to simplya.storyblok.com,
which is the default domain for assets; - add
/m
at the end of the URL; - move the filters from the beginning of your path to the end;
- if you are also using a custom domain to serve the assets stored in Storyblok, you need to forward the
accept
header from your CloudFront distribution. You can check the updates to the Custom Assets' Domain doc to update your configuration.
Examples
The URL of your asset is https://a.storyblok.com/f/39898/3310x2192/e4ec08624e/demo-image.jpeg
and you want to apply the filters /200x0/filters:format(png)
. The URL with the first version of the image service is:
https://img2.storyblok.com/200x0/filters:format(png)/f/39898/3310x2192/e4ec08624e/demo-image.jpeg
In the new version, after applying the three transformations from the list above, the URL will look like this:
https://a.storyblok.com/f/39898/3310x2192/e4ec08624e/demo-image.jpeg/m/200x0/filters:format(png)